How to Integrate Biometric Authentication in your iPhone Apps
- Mobile
- December 10, 2018
Apple iOS always believes in implementing the latest upgraded technology in their devices.
Last year, it launched the iPhone X and this year we saw the emergence of iPhone XR and iPhone XS with several new advanced features and functionalities. One of the popular functionalities is the introduction of the Touch ID and Face Id.
However, Apple has introduced the Touch ID long before and its available from the iPhone 5S models. This is a unique feature, which lets the user unlock their devices with their fingerprints.
Thus, it took the technology a one step further where users didn’t need to memorize the password key or the lock pattern.
Initially, the iOS developers found it difficult to authenticate the Touch ID in the iOS 7 version, but with the release of the iOS8, Apple successfully solved this issue as it offered the official support of API to use the apps.
So, let’s first start with the integration of the touch id authentication in the iOS app.
Creating a New Project
The first step would be creating a new project under the file menu and then choose the Single View Application. Then, click on the next button. You would get the interface something like in the figure below.
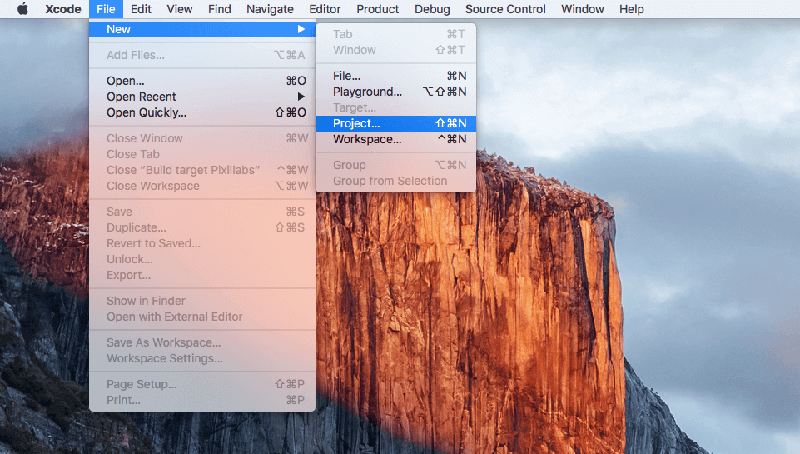
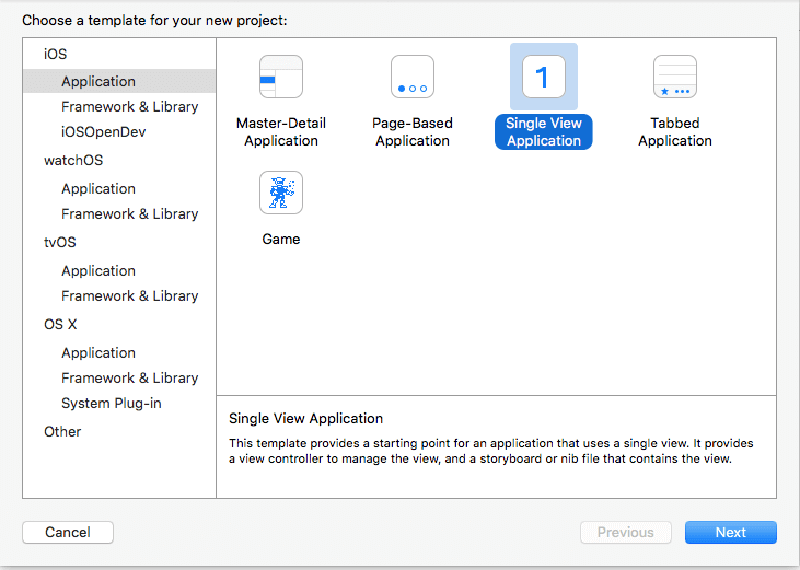
Naming your Project
In the next step, you need to name your project. For example, you can name it as Biometrics Authentication.
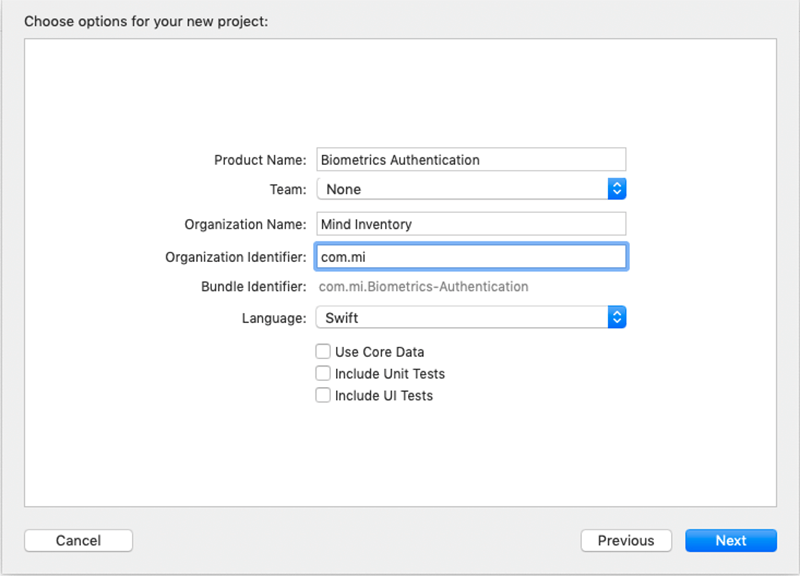
Upon the successful creation of the project, it’s time to import the Local Authentication in ViewController.swift.
Adding Privacy Permission for using of NSFaceIDUsageDescription
One needs to go for the configuration of the Face ID privacy statement in the Info.plist file statement of the project.
This is the privacy statement, which a user sees when the app wants to take permission to use the Face ID authentication. For the addition of this privacy statement, first, choose the Info.plist file in the project navigator panel and click on the + button that is found at the bottom of the list entry.
Next is choosing the Privacy – Face ID Usage Description from the desired outcome of the previous program. Now, add the description into the desired field as shown in the figure.

Going Through the Biometric Authentication Availability
But wait for a minute. Have you checked the biometric authentication availability? It’s a necessary step to follow because not all iOS devices have the facility of fingerprint scan and do not support the integration of touch id and facial id.
Therefore, you need to check the biometric authentication availability first. This is applicable for both integrating the touch and face id.
Checking the Accessibility of Biometric
It’s important for you to check the biometric availability before proceeding further. If your device can support the Biometric Sensor, you can think of implementing the fingerprint scan or the face id verification.
The following code will well indicate whether the device will support the Touch ID and the Face ID and also update the text on the authButton instance accordingly.
import UIKit
import LocalAuthentication
class ViewController: UIViewController {
// The first step would be to define few properties on the global level for your view controller
@IBOutlet weak var imgAuthenticate: UIImageView!
let context = LAContext()
var strAlertMessage = String()
var error: NSError?
override func viewDidLoad() {
super.viewDidLoad()
if context.canEvaluatePolicy(
LAPolicy.deviceOwnerAuthenticationWithBiometrics,
error: &error) {
if context.canEvaluatePolicy(.deviceOwnerAuthenticationWithBiometrics, error: &error) {
switch context.biometryType {
case .faceID:
self.strAlertMessage = "Set your face to authenticate"
self.imgAuthenticate.image = UIImage(named: "face")
break
case .touchID:
self.strAlertMessage = "Set your finger to authenticate"
self.imgAuthenticate.image = UIImage(named: "touch")
break
case .none:
print("none")
//description = "none"
break
}
}else {
// Device cannot use biometric authentication
if let err = error {
// calling error message function based on the error type
let strMessage = self.errorMessage(errorCode: err._code)
self.notifyUser("Error",
err: strMessage)
}
}
}else{
if let err = error {
let strMessage = self.errorMessage(errorCode: err._code)
self.notifyUser("Error",
err: strMessage)
}
}
}
Getting the Biometric Authentication from User
Once you are confirmed, that it would support the Touch Id, you can go with the following command:
@IBAction func authenticateUser(_ sender: Any) {
// Device can use biometric authentication
if context.canEvaluatePolicy(
LAPolicy.deviceOwnerAuthenticationWithBiometrics,
error: &error) {
context.evaluatePolicy(
.deviceOwnerAuthenticationWithBiometrics,
localizedReason: self.strAlertMessage,
reply: { [unowned self] (success, error) -> Void in
DispatchQueue.main.async {
if( success ) {
//Fingerprint recognized
// Do whatever action you want to perform
} else {
//If not recognized then
if let error = error {
let strMessage = self.errorMessage(errorCode: error._code)
print(strMessage)
}
}
}
})
}
}
Facing the Error Challenges During Integration
As an iOS developer, you must prepare beforehand to tackle the challenges related to errors while implementing the Touch Id authentication in your device. The error code may appear as:
// MARK: Get error message
func errorMessage(errorCode:Int) -> String{
var strMessage = ""
switch errorCode {
case LAError.authenticationFailed.rawValue:
strMessage = "Authentication Failed"
case LAError.userCancel.rawValue:
strMessage = "User Cancel"
case LAError.userFallback.rawValue:
strMessage = "User Fallback"
case LAError.systemCancel.rawValue:
strMessage = "System Cancel"
case LAError.passcodeNotSet.rawValue:
strMessage = "Passcode Not Set"
case LAError.biometryNotAvailable.rawValue:
strMessage = "TouchI DNot Available"
case LAError.biometryNotEnrolled.rawValue:
strMessage = "TouchID Not Enrolled"
case LAError.biometryLockout.rawValue:
strMessage = "TouchID Lockout"
case LAError.appCancel.rawValue:
strMessage = "App Cancel"
case LAError.invalidContext.rawValue:
strMessage = "Invalid Context"
default:
strMessage = "Some error found"
}
return strMessage
}
Now that the integration of Touch Id is over, let’s talk about the implementation of the Face ID. Till now we have sought the biometric authentication from the user. Here the next step would be the addition of the Face ID privacy statement.
The Application Testing using Face ID
Once you have integrated the Face ID, it’s time now to conduct some biometric authentication testing.
You can follow two methodologies here; the first is using the iOS devices manually where you get the biometric support. In the second process, you need to employ the simulator environment.
If you are opting for the second method to conduct the Face ID integration test, then the compilation and running the app on an iPhone X simulator becomes important.
When the app gets released, you need to choose the simulator’s hardware and then select the Face ID menu. However, it must be ensured that you have enabled the enrolled option. Now, tap on the authentication button in the app.
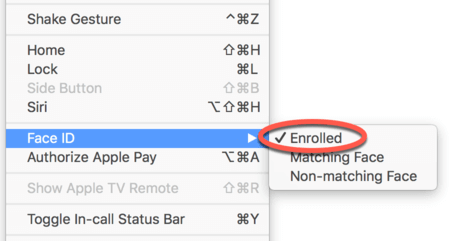
After the test is completed, you will get an option where the app would ask your permission whether you want to allow the biometric to use the Face ID. For the Face ID, it will be showing the privacy statement that was earlier displayed in the Info.plist file.
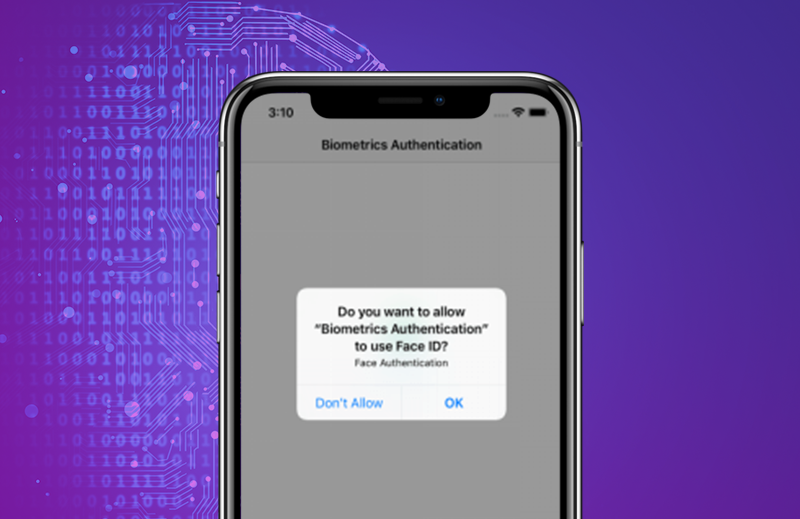
Once you allow access, you would come across a home screen where you can see the Authenticate button with Face ID icon and Fingerprint icon in case of Touch ID authentication.
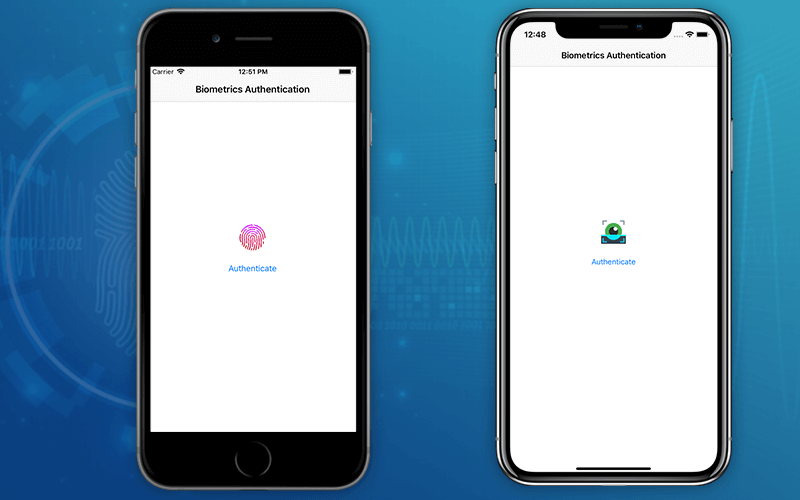
Once you click on the Authenticate button you will come across gray simulated Face ID panel :
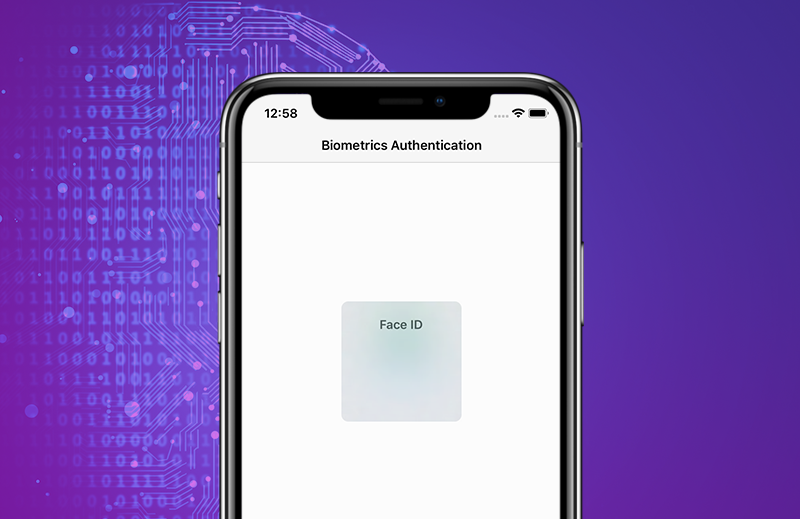
If you want to match the face, then choose the Hardware and correct Face ID and then the Matching Face Menu option.
In case of Touch ID, it will ask you in the popup to set a finger,
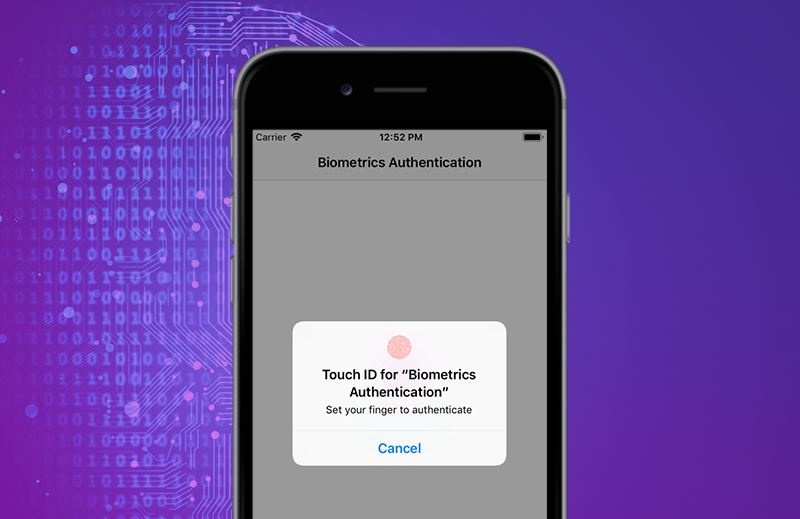
You would then redirected on the new page with the message that the authentication is successful. You can find the complete sample for this on the GitHub link added in the end.
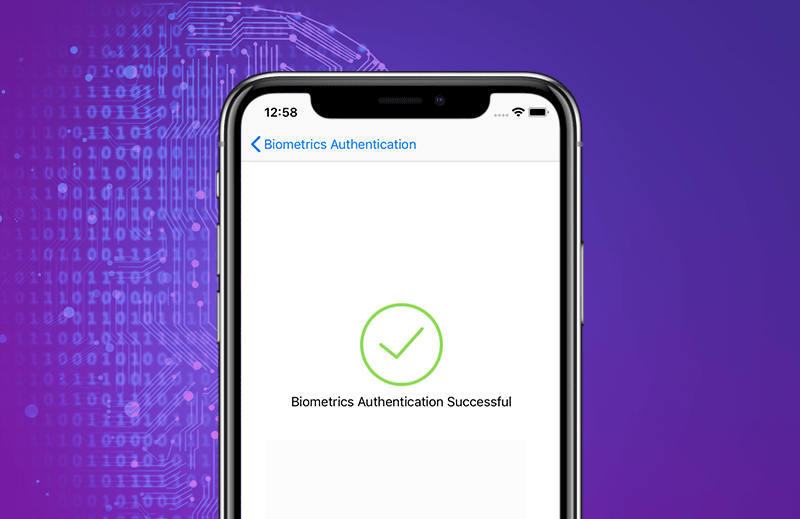
Now, You can repeat this process and select the Non matching category menu option this time to check if the authentication fails.
Conclusion
We can say that both Touch ID as well as the Face ID is one of the most useful and attractive features implemented in the recent few models of the iPhone.
As such, the users love it as they feel that their iPhone devices have become much more smart and secured. They don’t need to remember the password or the lock pattern to unlock the phone.
It should be noted that Touch ID was introduced with the launch of iOS 8 and the face recognition came along with the unveiling of iPhone X last year.
Our iOS app developers are highly experienced and skilled in integrating the touch id and face id. Get in touch with them today!
FAQs About Biometric Authentication
Biometric authentication is simply the security process to verify identity through unique characteristics of the human body. Biometric authentication systems store this data in order to verify a user’s identity.
There are two common types of biometric authentication in the iPhone app: Face ID and Touch ID.
There are several benefits of biometric authentication like accurate identification, secure, efficient, convenient, easily scalable, etc.